Creating Angular Applications with Firebase + Gemini
Mayra Rodriguez
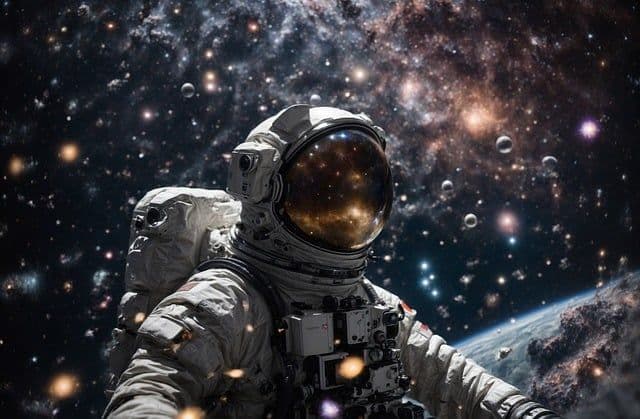
Creating Angular Applications with Firebase + Gemini
Introduction
In the world of web development, creating robust and scalable applications is a top priority. Angular, Firebase, and Gemini are powerful tools that, when combined, can significantly streamline your development process and enhance the functionality of your applications. This blog post will guide you through the steps of integrating Firebase and Gemini into your Angular applications.
What is Angular?
Angular is a platform and framework for building single-page client applications using HTML and TypeScript. Developed and maintained by Google, Angular provides a robust set of tools and best practices for building dynamic, responsive web applications.
What is Firebase?
Firebase, also developed by Google, is a platform that provides a suite of tools and services to help you develop high-quality apps, grow your user base, and earn more money. It offers backend services such as real-time databases, authentication, analytics, and cloud storage.
What is Gemini?
Gemini is a relatively newer tool that focuses on enhancing the development workflow with AI-powered code suggestions and integrations. It can be particularly useful for optimizing your code and improving overall productivity.
Setting Up Your Angular Project
- Install Angular CLI: First, ensure that you have the Angular CLI installed. If not, you can install it via npm:
npm install -g @angular/cli
- Create a New Angular Project: Use the CLI to create a new project:
ng new my-angular-app
cd my-angular-app
Integrating Firebase
- Set Up Firebase Project: Go to the Firebase Console, create a new project, and add a web app to your project.
- Install Firebase and AngularFire: In your Angular project directory, install Firebase and AngularFire:
npm install firebase @angular/fire
- Add Firebase Configuration: Copy the Firebase configuration details from your Firebase project settings and add them to your Angular project. Create a new file
src/environments/environment.ts
and add the following:
export const environment = {
production: false,
firebase: {
apiKey: "YOUR_API_KEY",
authDomain: "YOUR_PROJECT_ID.firebaseapp.com",
databaseURL: "https://YOUR_PROJECT_ID.firebaseio.com",
projectId: "YOUR_PROJECT_ID",
storageBucket: "YOUR_PROJECT_ID.appspot.com",
messagingSenderId: "YOUR_MESSAGING_SENDER_ID",
appId: "YOUR_APP_ID"
}
};
- Initialize Firebase in Your App: Modify
src/app/app.module.ts
to include AngularFire modules:
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { AngularFireModule } from '@angular/fire';
import { AngularFireDatabaseModule } from '@angular/fire/database';
import { environment } from '../environments/environment';
@NgModule({
declarations: [
// your components here
],
imports: [
BrowserModule,
AngularFireModule.initializeApp(environment.firebase),
AngularFireDatabaseModule
],
providers: [],
bootstrap: [/* your main component */]
})
export class AppModule { }
Integrating Gemini
- Sign Up for Gemini: Visit the Gemini website and sign up for an account. Follow the instructions to integrate Gemini into your development environment.
- Configure Gemini: Configure Gemini in your project by following the integration guide provided by Gemini. This usually involves installing a plugin or extension in your code editor (such as VSCode) and logging in with your Gemini account.
- Using Gemini for Code Suggestions: As you write your Angular code, Gemini will provide AI-powered code suggestions and improvements. This can help you write more efficient and error-free code.
Building Your Application
With Firebase and Gemini integrated, you can now start building your Angular application. Use Firebase for your backend needs, such as real-time database and authentication, and leverage Gemini's AI capabilities to enhance your coding efficiency.
Conclusion
Integrating Firebase and Gemini into your Angular applications can significantly boost your development process. Firebase provides a powerful backend solution, while Gemini enhances your coding workflow with AI-powered suggestions. Together, they create a robust environment for building scalable and efficient web applications. Happy coding!